Java + AppleScript
Java 6 introduced the ability to run AppleScript from within Java. For a long time I considered this a solution in need of a problem. However I’ve been working recently on making it easier to find and report hand history problems in Poker Copilot. With a little bit of AppleScript I can open the Mail application, create a message, and attach a file. Java supplies the recipient’s email address, the subject, the body of the message, and the attachment. Put it together and it looks like the following:
import javax.script.ScriptEngineManager;
import javax.script.ScriptException;
public class ApplescriptEmailer {
public void createEmailWithAttachment(String recipient, String subject, String content, String attachmentFilename) throws ScriptException {
final String script =
"tell application \"Mail\"\n" +
" set theMessage to make new outgoing message with properties {visible:true, subject:\"" + subject + "\", content:\"" + content + "\"}\n" +
" tell theMessage\n" +
" make new to recipient at end of to recipients with properties {address:\"" + recipient + "\"}\n" +
" end tell\n" +
" tell content of theMessage\n" +
" make new attachment with properties {file name:\"" + attachmentFilename + "\"} at after last paragraph\n" +
" end tell\n" +
" activate\n" +
"end tell";
new ScriptEngineManager().getEngineByName("AppleScript").eval(script);
}
public static void main(String[] args) throws ScriptException {
final String filename = "/Users/steve/Documents/problem file.txt";
final String subject = "Poker Copilot Problem Hand History File";
final String content = "Dear Poker Copilot Support\n\nPoker Copilot is having problems with the attached hand history file.\n\n";
final String recipient = "support@pokercopilot.com";
new ApplescriptEmailer().createEmailWithAttachment(recipient, subject, content, filename);
}
}
Here’s the result:
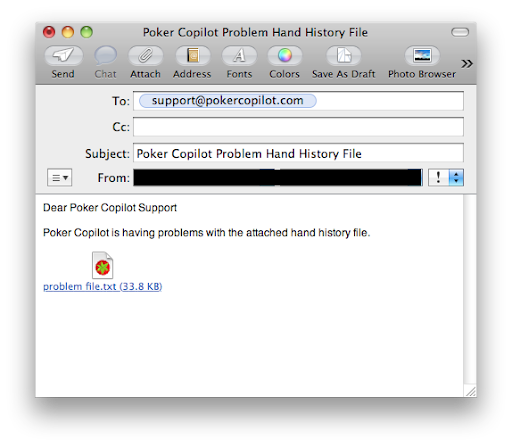